Recently, a client asked my team to build a simple React app with Google Firebase for the backend. While I wasn’t familiar with the Cloud Firestore environment, I figured it would be fine—it was a Google product, right? I was also fresh out of Lambda School’s lessons on backend systems and feeling so fly about the databases I built in PostgreSQL and SQLite. Surely, this wouldn’t be terribly different, right? I’d pick it up as I go. Right?
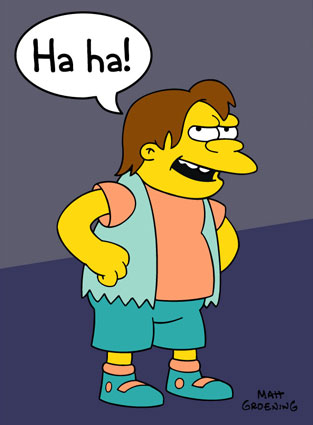
Our client needed a regular React app hooked to Firebase. As I started researching how to do this, I found a few pain points:
- Adding Firebase Firestore to React—it was a bit confusing to this n00b
- Firebase and React, JUST React: Most examples of Firebase and React online were Firebase with React Native (for mobile excellence) or Redux (for overachievers). My team did consider those options briefly, but ultimately, they were not in scope
- Testing the database with Jest (and only Jest) was in short supply. Most tests I found involved Enzyme, which, again, was not in scope
- Moving Firebase from test to production was an epic voyage
For those of you who want to skip ahead to the good stuff, here’s the GitHub repo
—I understand, you’re probably on deadline and this is the millionth blog you’ve found. It’s OK.
In the next few posts, we’ll address all of these issues. In this one, we’re going to do a “For Dummies” on how to hook up Firebase Firestore to a React app. Don’t feel bad if you’re struggling with this—I did too, that’s why we’re here today.
So, I’m assuming you had no problem setting up Create React App and creating a new project (your database) in Firebase. If not, Net Ninja’s Firebase Firestore tutorials cover both really well, and Create React App’s documentation is also easy to follow.
To connect React and Firebase you need to do a bit of back-and-forth work in your React app and your Firebase project.
Start with getting your materials ready. You will need
1. Your React app files open in a code editor (I use VSC)
2. An open terminal cd-ed into your React app’s src file
3. Your Firebase project open in a browser
The first step is to add Firebase to your dependencies (in your package.json file). In a terminal, CD into your React app’s src file you can do one of the following in a terminal:
npm install -g firebase *
yarn add firebase
IN FIREBASE
Once you’ve installed Firebase in your React app, go to your Firebase console to set it up.
1) Open your project
2) Click on the web icon to create </>
3) Create an app nickname (it can be named anything), do not select “Firebase Hosting” (you can do this later) and FINALLY, click “Register App” to continue
4) Copy the code—you will be using it in your React file**. Since you have a different project than mine, your code will look a bit different, but should have the same/very similar components.
After you’ve copied that text, click the “Continue to Console” button and head back to your React file.
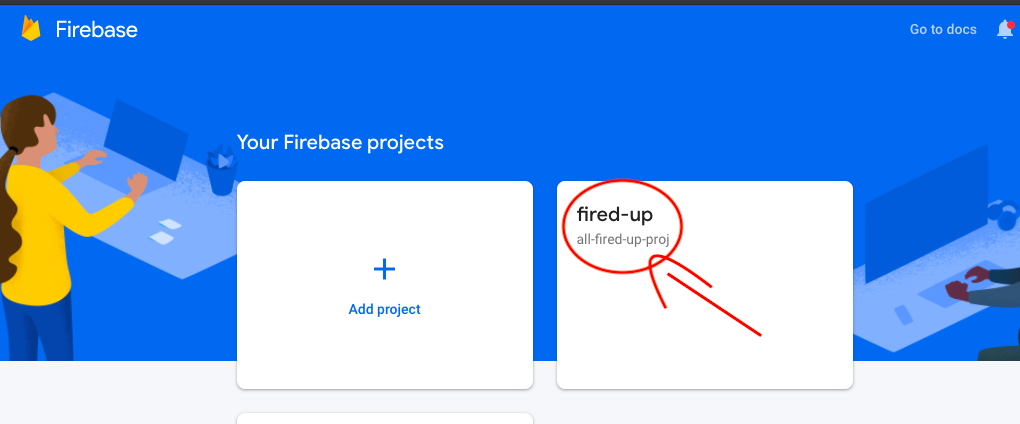
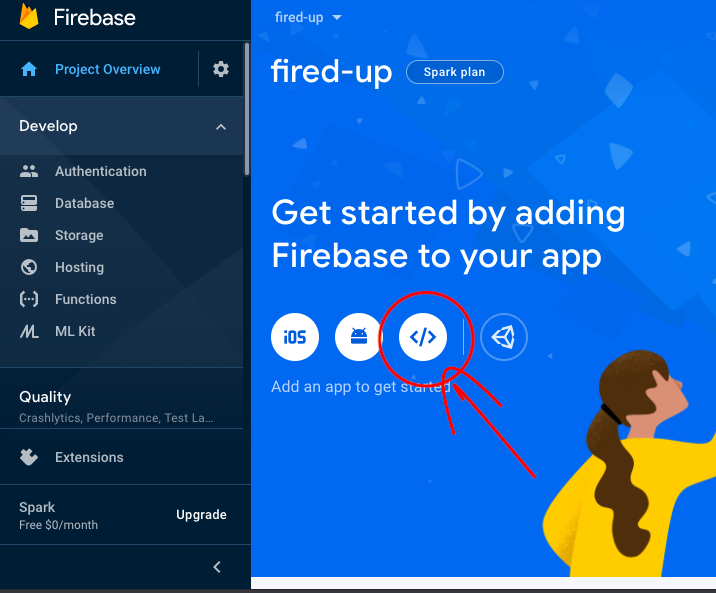
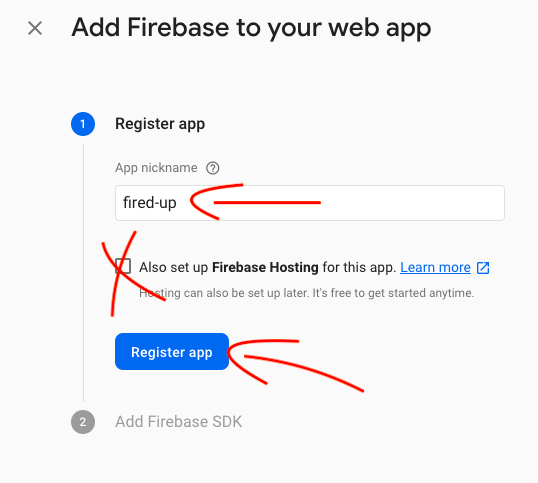
DO NOT SELECT FIREBASE HOSTING (FOR NOW)
CLICK REGISTER APP
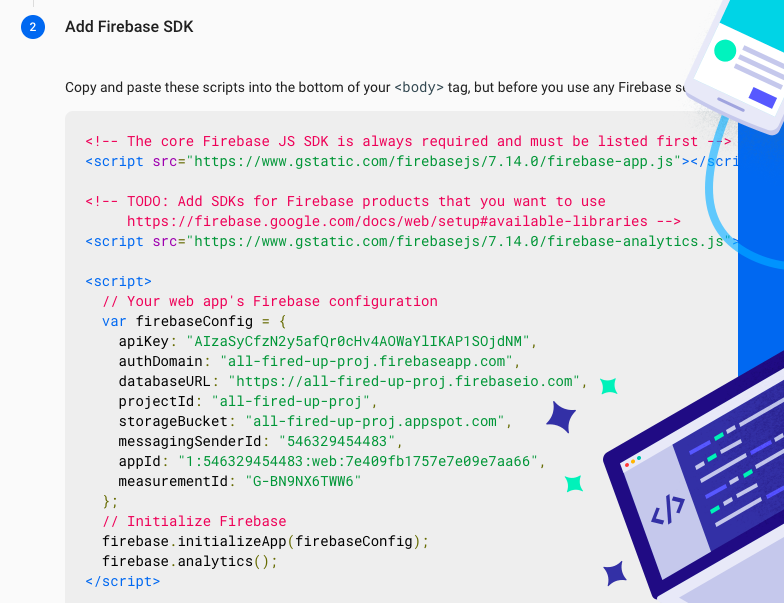
IN REACT
Back in your React app’s src file, navigate to the index.html page in your public folder, src > public > index.html. It should look something like this. (You’re going to be putting things into the <body> section):
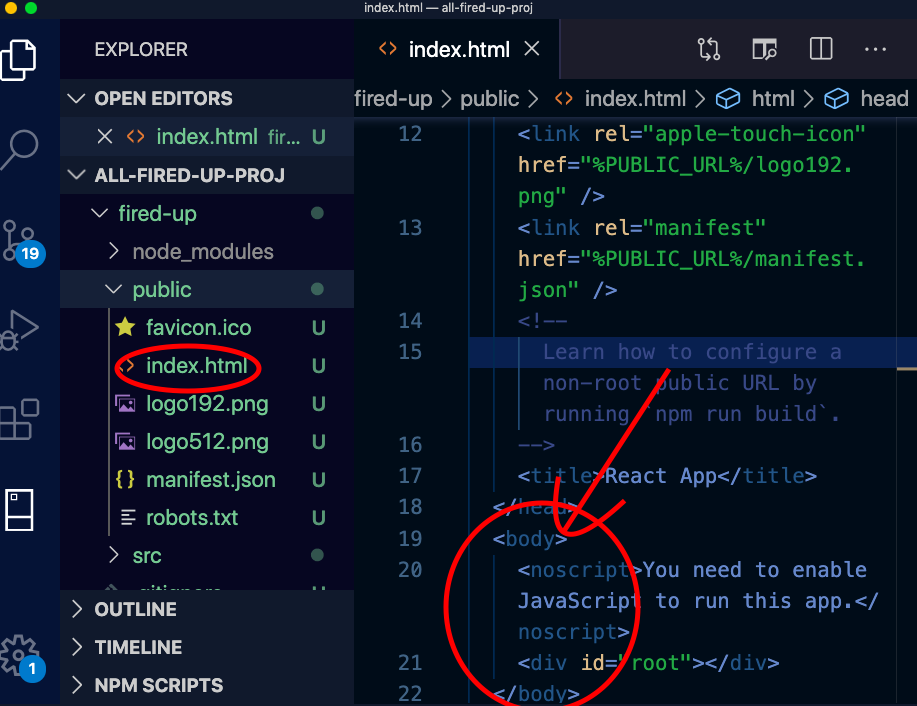
Remember that code you copied from Firebase? Paste that bad boy into the <body> section of your index.html file. It should look something like this when you’re done:
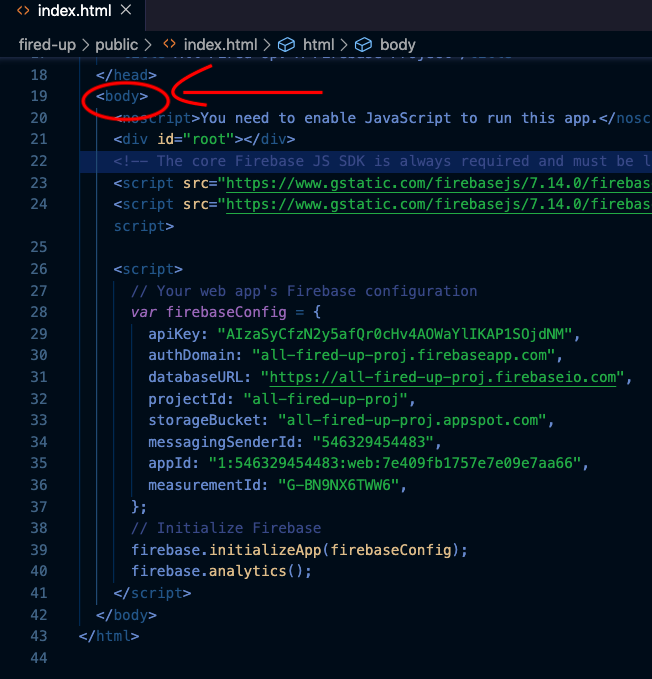
Last step. Almost there!
In your React app, create a firebase.js file in your React app’s src folder and add that Firebase SDK content. (You can put this in a config folder, if you like.) Don’t forget, you have import firebase dependencies!
It should look like this when you’re done:
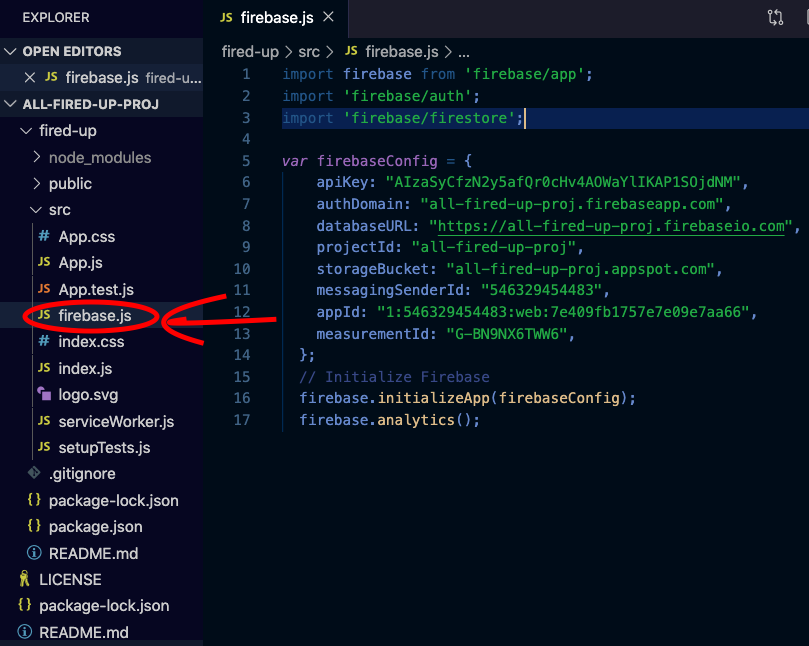
And with that, you are done setting up Firebase in your React app! Stay tuned for Part 2, where we are going to implement Firebase CRUD-ily into the React app.
FIELD NOTES
When you initially set up your Firebase project, you will be encouraged to develop in “test” mode. We’ll go into how to deploy that to production with Google hosting further down the line.
**You may not need to use what is in the Firebase SDK in index.html if you are hosting the website outside of Google — say, with AWS, Heroku, etc. What this means is that the values inside var firebaseConfig {}; (the stuff on the other side of apiKey:, authDomain:, etc) will have to match what you get from your host, and ALSO be placed in a dotenv file. I’ll show you an example of this, too, don’t worry.